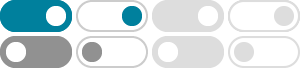
wait - How do I make a delay in Java? - Stack Overflow
If you want to pause then use java.util.concurrent.TimeUnit: TimeUnit.SECONDS.sleep(1); To sleep for one second or. TimeUnit.MINUTES.sleep(1); To sleep for a minute. As this is a loop, this presents an inherent problem - drift. Every time you run code and then sleep you will be drifting a little bit from running, say, every second.
What is the JavaScript version of sleep()? - Stack Overflow
Jun 4, 2009 · Here you go. As the code says, don't be a bad developer and use this on websites. It's a development utility function. // Basic sleep function based on ms. // DO NOT USE ON PUBLIC FACING WEBSITES. function sleep(ms) { var unixtime_ms = new Date().getTime(); while(new Date().getTime() < unixtime_ms + ms) {} }
How to make java delay for a few seconds? - Stack Overflow
Apr 25, 2014 · Java Thread Sleep important points. It always pause the current thread execution. Thread sleep doesn’t lose any monitors or locks current thread has acquired. Any other thread can interrupt the current thread in sleep, in that case InterruptedException is thrown.
Is there a sleep function in JavaScript? - Stack Overflow
Jul 17, 2009 · The answers are not good, the simplest and best answer is: function sleep(ms) { return new Promise ...
How to pause my Java program for 2 seconds - Stack Overflow
Apr 20, 2017 · Thread.sleep(2000); or. java.util.concurrent.TimeUnit.SECONDS.sleep(2); Please note that both of these methods throw InterruptedException, which is a checked Exception, So you will have to catch that or declare in the method. Edit: After Catching the exception, your code will look like this:
Make a java program sleep without threading - Stack Overflow
Nov 15, 2016 · Is there a way I can make the program sleep for a few milliseconds after it has done the calculations so that the upload takes place properly. (Like in other languages sleep or wait function) Thread.sleep(milliseconds) is a public static method that will work with single threaded programs as well. Something like the following is a typical pattern:
java - Sleep and check until condition is true - Stack Overflow
Is there a library in Java that does the following? A thread should repeatedly sleep for x milliseconds until a condition becomes true or the max time is reached. This situation mostly occurs when a test waits for some condition to become true. …
Sleep () in Android Java - Stack Overflow
Oct 24, 2018 · Thread.sleep(timeInMills); or. SystemClock.sleep(timeInMills); SystemClock.sleep(milliseconds) is a utility function very similar to Thread.sleep(milliseconds), but it ignores InterruptedException. Use this function for delays if you do not use Thread.interrupt(), as it will preserve the interrupted state of the thread.
java - How Thread.sleep () works internally - Stack Overflow
Jun 23, 2014 · The Thread.sleep() method essentially interacts with the thread scheduler to put the current thread into a wait state for the required interval. The thread however does not lose ownership of any monitors. In order to allow interruption, the implementation may not actually use the explicit sleep function that most OS's provide.
How to use thread.sleep () properly in Java? - Stack Overflow
Mar 18, 2015 · try { Thread.sleep((int)Millis); } catch (InterruptedException e) { } Apparently, an empty catch clause is not a good idea. If the thread is interrupted, the sleep period will not be finished completely. I am wondering if there's no logic in my code to interrupt the thread, could JVM interrupt a thread which is sleeping?