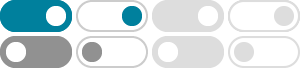
Triangle classifier in Java - Code Review Stack Exchange
Sep 14, 2017 · Triangle has 3 instance variables: int side1, side2, side3; The class Triangle must include the following constructors and methods: (If your class does not contain any of the following methods, points will be deducted). public Triangle (int s1, int s2, int s3) - Sets up a triangle with the specified side lengths. private int largest()
Modeling a Triangle, with a utility method to classify it
Jul 15, 2015 · If you have a valid triangle there is no reason to keep the Type.INVALID type; The triangle classification logic is put into the Type enum itself for a better encapsulation; When uncertain I tend to make the access modifiers as private as possible
Determine if a triangle is equilateral, isosceles, or scalene
Jul 25, 2019 · import unittest from triangle import equilateral, isosceles, scalene class TestEquilateralTriangle ...
Select the type of triangle based on the input
Mar 12, 2023 · The logic can be simplified if we sort the three lengths before classifying the triangle. Then the test for equilaterality becomes simply side[0] == side[2] , for example, and for right-angle is a single equality test, rather than ORing together three different ones.
Determining triangle type from three integer inputs
Oct 28, 2015 · public enum TriangleType { ISOSCELES, EQUILATERAL, SCALENE; public static TriangleType ofPotentialTriangle(PotentialTriangle triangle) { throwIf(triangle.isAnySideTooShort(), "Length of sides cannot be equal to or less than zero"); throwIf(triangle.violatesTriangleInequality(), "Sum of any two sides must be larger than the …
python - Determine whether three sides form a valid triangle, and ...
Jul 24, 2019 · If we enter an invalid triangle (e.g. 1, 2, 4), the program reports that it's scalene, before telling us that it's not a valid triangle. That's a contradiction - if it's not a triangle, it cannot be a scalene triangle! I recommend performing the is_valid_triangle() test first, and only continuing to classify the triangle if the test is successful.
Triangle class implementation - Code Review Stack Exchange
Jul 11, 2016 · Stack Exchange Network. Stack Exchange network consists of 183 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Project Euler #12 - first triangle number with more than 500 divisors
Dec 27, 2014 · The solution to the problem "the first number that has 500 divisors" results in a number somewhat larger than 75,000,000. That is somewhat after the 12-thousandth triangle number. So, in this case, you are looping through 12,000 times, and that's the same regardless of whether you use your old, or your new getDivisorCount method.
Pretty-print Pascal's triangle - Code Review Stack Exchange
Mar 2, 2014 · Enter the number of rows you want in your Pascal`s Triangle: 16 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1 1 5 10 10 5 ...
javascript - On right click draw triangle to the canvas - Code …
Sep 27, 2017 · stage and triangle do not need to be in global scope. triangle is only used in the one function so define it there. Use const rather than var if you do not intend to modify a variable. Don't let lines get too long. 80 character is the usual limit. If a line gets longer than that break it into multiple lines. Right click will bring up the ...