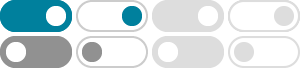
python - Sorting a set of values - Stack Overflow
Jul 3, 2013 · s = {10, 39, 3} s_list = list(s) s_list.sort() # sort in-place # [3, 10, 39] Since Python 3.7, dicts keep insertion order. So if you want to order a set but still want to be able to do membership checks in constant time, you can create a dictionary from the sorted list using dict.fromkeys() (the values are None by default).
python - How do I sort a list of objects based on an attribute of …
Feb 27, 2023 · The second sort accesses each object only once to extract its count value, and then it performs a simple numerical sort which is highly optimized. A more fair comparison would be longList2.sort(cmp = cmp). I tried this out and it performed nearly the same as .sort(). (Also: note that the "cmp" sort parameter was removed in Python 3.) –
python - Sorting the order of bars in pandas/matplotlib bar plots ...
What is the Pythonic/pandas way of sorting 'levels' within a column in pandas to give a specific ordering of bars in bar plot. For example, given: import pandas as pd df = pd.DataFrame({ 'gro...
Python list sort in descending order - Stack Overflow
Mar 16, 2023 · This is a strange answer because you do the sorting in-place but then the reversing out-of-place. If there is another variable aliasing the original list, its value afterwards will not have the elements in their original order, nor in descending order; the alias will point at a list sorted in ascending order.
python - How to sort pandas dataframe by one column - Stack …
If you want to sort by two columns, pass a list of column labels to sort_values with the column labels ordered according to sort priority. If you use df.sort_values(['2', '0']), the result would be sorted by column 2 then column 0. Granted, this does not really make sense for this example because each value in df['2'] is unique.
python - Sort an enumerated list by the value - Stack Overflow
Jul 10, 2017 · Python sorting key function supporting tuples and ... Now suppose I want to sort this list by the index 1 ...
python - How to sort a 2D list? - Stack Overflow
I want to sort this in descending order of the second column. I tried built-in sorted() function in python, but it gives me 'TypeError' : 'float' object is unsubscriptable . python
How do you get a directory listing sorted by creation date in …
Here's a one-liner: import os import time from pprint import pprint pprint([(x[0], time.ctime(x[1].st_ctime)) for x in sorted([(fn, os.stat(fn)) for fn in os.listdir ...
python - How to sort objects by multiple keys? - Stack Overflow
items.sort(key=operator.itemgetter('age'), reverse=True) items.sort(key=operator.itemgetter('name')) Note how the items are first sorted by the "lesser" attribute age (descending), then by the "major" attribute name , leading to the correct final order.
python - case-insensitive list sorting, without lowercasing the …
Apr 22, 2012 · In Python 2 it works for a mix of normal and unicode strings, since values of the two types can be compared with each other. Python 3 doesn't work like that, though: you can't compare a byte string and a unicode string, so in Python 3 you should do the sane thing and only sort lists of one type of string.