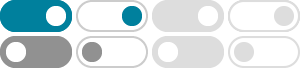
Java For Each Loop - W3Schools
There is also a "for-each" loop, which is used exclusively to loop through elements in an array (or other data sets): Syntax for ( type variableName : arrayName ) { // code block to be executed }
For-Each Loop in Java - GeeksforGeeks
Mar 18, 2025 · The for-each loop in Java, introduced in Java 5, simplifies iteration over arrays and collections, enhancing readability but has limitations such as inability to modify elements directly, lack of index access, and potential performance overhead compared to traditional loops.
foreach - In detail, how does the 'for each' loop work in Java?
The following is the very latest way of using a for each loop in Java 8 (loop a List with forEach + lambda expression or method reference). Lambda // Output: A,B,C,D,E items.forEach(item->System.out.println(item));
Java forEach() with Examples - HowToDoInJava
The forEach() method in Java is a utility function to iterate over a Collection (list, set or map) or Stream. The forEach() performs a given Consumer action on each item in the Collection. List<String> list = Arrays.asList("Alex", "Brian", "Charles"); list.forEach(System.out::println);
Guide to the Java forEach - Baeldung
Feb 15, 2025 · Introduced in Java 8, the forEach() method provides programmers with a concise way to iterate over a collection. In this tutorial, we’ll see how to use the forEach() method with collections, what kind of argument it takes, and how this loop differs from the enhanced for-loop.
Java 8 Iterable.forEach() vs foreach loop - Stack Overflow
May 19, 2013 · With Stream.forEach (), you can't update local variables since their capture makes them final, which means you can have a stateful behaviour in the forEach lambda (unless you are prepared for some ugliness such as using class state variables). The better practice is …
The for-each Loop in Java - Baeldung
Jan 8, 2024 · In this tutorial, we’ll discuss the for -each loop in Java along with its syntax, working, and code examples. Finally, we’ll understand its benefits and drawbacks. 2. Simple for Loop. The simple for loop in Java essentially has three parts – initialization, boolean condition & …
Java 8 forEach with List, Set and Map Examples - Java Guides
In this post, we will learn how to forEach () method to iterate over collections, maps, and streams. Let's use normal for-loop to loop a List. final List < Person > items = new ArrayList < > (); items. add(new Person (100, "Ramesh")); items. add(new Person (100, "A")); items. add(new Person (100, "B")); items. add(new Person (100, "C"));
How to perform forEach loop in Java - Stack Overflow
Mar 24, 2014 · Since Java 5, you can use the enhanced for loop for this. Assume you have (say) a List<Thingy> in the variable thingies. The enhanced for loop looks like this: for (Thingy t : thingies) { // ... } As of Java 8, there's an actual forEach method on iterables that accepts a lambda function: thingies.forEach((Thingy t) -> { /* ...your code here...
Java 8 forEach () loop complete guide with examples
February 25, 2021 - Learn how to use a forEach() loop in java 8 syntax to iterate or loop through a list, map, stream with examples and explanation.